[View] [Edit] [Lock] [References] [Attachments] [History] [Home] [Changes] [Search] [Help]
[U8] iOS UI Framework
The iOS UI is based on Windows and Views which are used to present application content on the screen.
Windows do not have any visible content but provide a basic container for the views. Views define a portion of a window that will be filled with some content. Views can also be used to organize and manage other views.
Every iOS application has at least one window and one view for presenting its content.
Views
A view is an instance of the UIView class (or one of its subclasses) and manages a rectangular area in an application window. Views are responsible for drawing content, handling multitouch events, and managing the layout of any subviews. In the view hierarchy, parent views are responsible for positioning and sizing their child views dynamically.
In general a developer uses several views as building blocks to build a view hierarchy (and corrdinate it through view controllers, see section "iOS UI Elements" below)
As expected most operations on parent views are chained to child views (resizing, events, etc). Each superview stores its subviews in an ordered array and the order in that array also affects the visibility of each child.
Because view objects are the main way the application interacts with the user, they have many responsibilities:
- Layout and subview management
- A view defines its own default resizing behaviors in relation to its parent view
- A view can manage a list of subviews
- A view can override the size and position of its subviews as needed
- A view can convert points in its coordinate system to the coordinate systems of other views or the window
- Drawing and animation
- A view draws content in its rectangular area.
- Some view properties can be animated to new values
- Event handling
- A view can receive touch events.
- A view participates in the responder chain
Layers
Every view is backed by a layer object (usually an instance of the CALayer class), which manages the backing store for the view and handles view-related animations. A layer can be accessed from that view’s layer property.
Most operations on a view should be through the UIView interface but when more control is needed over the rendering or animation behavior of a view, operations are done through its layer instead.
View Life Cycle
A View uses on-demand drawing model for presenting content. A snapshot is captured on the view's first drawing so it's reusable. When the content is changed the system is notified and the view repeats the process of drawing itself and capturing a snapshot of the new results.
When the contents of your view change you invalidate the view using either the setNeedsDisplay or setNeedsDisplayInRect: method. But before that you need to render your view’s content where the actual drawing process varies depending on the view and its configuration. System views typically implement private drawing methods to render their content. Those same system views often expose interfaces that you can use to configure the view’s actual appearance. For custom UIView subclasses, you typically override the drawRect: method of your view and use that method to draw your view’s content.
Windows
A window is an instance of the UIWindow class and handles the overall presentation of the application’s user interface. Windows work with views (and their owning view controllers) to manage interactions with, and changes to, the visible view hierarchy. Every application has at least one window that displays the application’s UI.
A window object has several responsibilities:
- It contains the application’s visible content
- It plays a key role in the delivery of touch events to the views and other application objects
- It works with the application’s view controllers to facilitate orientation changes
In iOS, windows do not have title bars, close boxes, or any other visual goodies. A window is always just a blank container for one or more views.
Most iOS applications create and use only one window during their lifetime. This window spans the entire main screen of the device and is loaded from the application’s main nib file (or created programmatically) early in the life of the application.
Task involving Windows
In general the only time the application interacts with its window is when it creates the window at startup. However, you can use your application’s window object to perform a few application-related tasks:
- Use the window object to convert points and rectangles to or from the window’s local coordinate system
- Use window notifications to track window-related changes
Animations
Animations provide users with visible feedback about changes to the view hierarchy. The system defines standard animations for presenting modal views and transitioning between different groups of views. Many attributes of a view can also be animated directly.
External Representation
Interface Builder is an application used to graphically construct and configure your application’s windows and views. Using Interface Builder the assembled views are placed in a nib file (a resource file). When nib file is loaded at runtime, the objects inside it are reconstituted into actual objects.
This is an interesting starting point for researching programmatic UI building in coco8.
iOS UI Elements
The UI elements fall into four broad categories:
- Bars: contain contextual information that tell users where they are and controls that help users navigate or initiate actions
- Content views: contain app-specific content and can enable behaviors such as scrolling, insertion, deletion, and rearrangement of items
- Controls: perform actions or display information
- Temporary views: appear briefly to give users important information or additional choices and functionality
A UI element is a type of view because it inherits from UIView. A view knows how to draw itself onscreen, and it knows when a user touches within its bounds. Controls (such as buttons and sliders), content views (such as collection views and table views), and temporary views (such as alerts and action sheets) are all types of views.
To manage a set or hierarchy of views in your app, you typically use a view controller. A view controller coordinates the display of views, implements the functionality behind user interactions, and can manage transitions from one screen to another. For example, Settings uses a navigation controller to display its hierarchy of views.
Here’s an example of how views and view controllers can combine to present the UI of an iOS app:
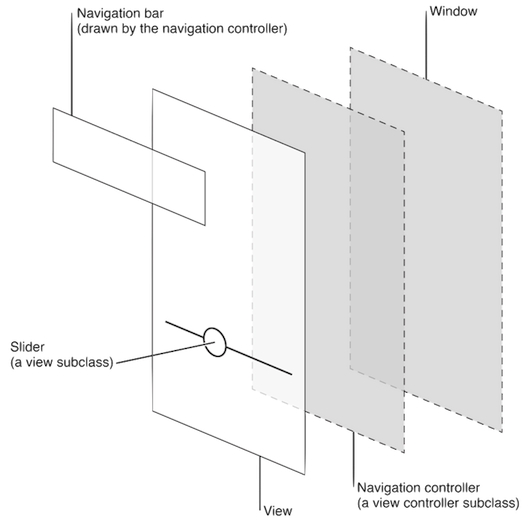
For a detailed description of UI elements see this page.